Serial Date
function serialdate(pdate)
' Parameter pdate is a date variable
' returned value is YYYYMMDD HH24MI
' uses function leadzero fill in leading zero
DIM tstr
tstr = year(pdate) & leadzero(month(pdate)) & leadzero(day(pdate)) & _
" " & leadzero(hour(pdate)) & leadzero(minute(pdate))
serialdate = tstr
end function
CheckBox
'==================================================================
' Subroutine to create a checkbox and decide if it should be selected or not
'==================================================================
Sub getCheck(cInput, cVal)
' Parameters are as follows:
' cInput - checkbox name
' cVal - status of checkbox (on or not)
Response.Write "<input type=""checkbox"" name="""
Response.Write cInput
Response.Write """"
if (cVal = "on") then
Response.Write " checked"
end if
Response.Write ">"
End Sub
To use above, you may create a checkbox as follows:
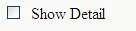
Check Buffer then flush the buffer
'==================================================================
' For checking the buffer count to decide if a response.flush is required
'==================================================================
function bufCheck(tCount)
tCount = tCount + 1
if (tCount > BuffLim) then
response.flush
bufCheck = 0
else
bufCheck = tCount
end if
end function
To use the above, declare a counter and a bufferlimit:
Dim cnt
Dim BuffLimit
BuffLimit = 50 'or whatever number you want
and then increment each time you do a response.write and then call the routine as follows:
cnt = cnt + 1
bufCheck(cnt)
This will reduce the amount of memory used on the server by flushing the buffer to the client once the limit is hit, otherwise it will wait until the asp is fully executed before sending the content to the client.
' =============================================================================
' Function to pad the string so that it has the length of tlen
' =============================================================================
function sp_pad(tString, tlen)
sp_pad = tString + space(tlen - len(tString))
end function
Radio Button
'============================
' For setting default value
'============================
sub defval(pfld, pval)
if pfld = "" then
pfld = pval
end if
end sub
'==================================================================
' check to see if the word checked should be written - used in conjunction
' with a radiobutton
'==================================================================
sub checked(pVar, pVal)
if pVar = pVal then
Response.Write("checked")
end if
end sub
Example of use is as follows:
<%
Dim cSel
cSel = request.form("carType")
defval cSel, "saloon"
%>
<input type="Radio" name="carType" value="saloon" <% checked cSel, "saloon" %>> Saloon
<input type="Radio" name="carType" value="4wd" <% checked cSel, "4wd" %>> 4 Wheel Drive
The above will look like this:

No comments:
Post a Comment